A slider is a control with a handle which can be pulled back and forth to change the value.
On Windows, the track bar control is used.
Slider events are handled in the same way as a scrollbar.
Styles
This class supports the following styles:
- wxSL_HORIZONTAL:
Displays the slider horizontally (this is the default).
- wxSL_VERTICAL:
Displays the slider vertically.
- wxSL_AUTOTICKS:
Displays tick marks. Windows only.
- wxSL_MIN_MAX_LABELS:
Displays minimum, maximum labels (new since wxWidgets 2.9.1).
- wxSL_VALUE_LABEL:
Displays value label (new since wxWidgets 2.9.1).
- wxSL_LABELS:
Displays minimum, maximum and value labels (same as wxSL_VALUE_LABEL and wxSL_MIN_MAX_LABELS together).
- wxSL_LEFT:
Displays ticks on the left and forces the slider to be vertical.
- wxSL_RIGHT:
Displays ticks on the right and forces the slider to be vertical.
- wxSL_TOP:
Displays ticks on the top.
- wxSL_BOTTOM:
Displays ticks on the bottom (this is the default).
- wxSL_SELRANGE:
Allows the user to select a range on the slider. Windows only.
- wxSL_INVERSE:
Inverses the minimum and maximum endpoints on the slider. Not compatible with wxSL_SELRANGE.
Notice that wxSL_LEFT
, wxSL_TOP
, wxSL_RIGHT
and wxSL_BOTTOM
specify the position of the slider ticks in MSW implementation and that the slider labels, if any, are positioned on the opposite side. So, to have a label on the left side of a vertical slider, wxSL_RIGHT must be used (or none of these styles at all should be specified as left and top are default positions for the vertical and horizontal sliders respectively).
Events emitted by this class
The following event handler macros redirect the events to member function handlers 'func' with prototypes like:
Event macros for events emitted by this class:
You can use EVT_COMMAND_SCROLL... macros with window IDs for when intercepting scroll events from controls, or EVT_SCROLL... macros without window IDs for intercepting scroll events from the receiving window -- except for this, the macros behave exactly the same.
- EVT_SCROLL(func):
Process all scroll events.
- EVT_SCROLL_TOP(func):
Process wxEVT_SCROLL_TOP
scroll-to-top events (minimum position).
- EVT_SCROLL_BOTTOM(func):
Process wxEVT_SCROLL_BOTTOM
scroll-to-bottom events (maximum position).
- EVT_SCROLL_LINEUP(func):
Process wxEVT_SCROLL_LINEUP
line up events.
- EVT_SCROLL_LINEDOWN(func):
Process wxEVT_SCROLL_LINEDOWN
line down events.
- EVT_SCROLL_PAGEUP(func):
Process wxEVT_SCROLL_PAGEUP
page up events.
- EVT_SCROLL_PAGEDOWN(func):
Process wxEVT_SCROLL_PAGEDOWN
page down events.
- EVT_SCROLL_THUMBTRACK(func):
Process wxEVT_SCROLL_THUMBTRACK
thumbtrack events (frequent events sent as the user drags the thumbtrack).
- EVT_SCROLL_THUMBRELEASE(func):
Process wxEVT_SCROLL_THUMBRELEASE
thumb release events.
- EVT_SCROLL_CHANGED(func):
Process wxEVT_SCROLL_CHANGED
end of scrolling events (MSW only).
- EVT_COMMAND_SCROLL(id, func):
Process all scroll events.
- EVT_COMMAND_SCROLL_TOP(id, func):
Process wxEVT_SCROLL_TOP
scroll-to-top events (minimum position).
- EVT_COMMAND_SCROLL_BOTTOM(id, func):
Process wxEVT_SCROLL_BOTTOM
scroll-to-bottom events (maximum position).
- EVT_COMMAND_SCROLL_LINEUP(id, func):
Process wxEVT_SCROLL_LINEUP
line up events.
- EVT_COMMAND_SCROLL_LINEDOWN(id, func):
Process wxEVT_SCROLL_LINEDOWN
line down events.
- EVT_COMMAND_SCROLL_PAGEUP(id, func):
Process wxEVT_SCROLL_PAGEUP
page up events.
- EVT_COMMAND_SCROLL_PAGEDOWN(id, func):
Process wxEVT_SCROLL_PAGEDOWN
page down events.
- EVT_COMMAND_SCROLL_THUMBTRACK(id, func):
Process wxEVT_SCROLL_THUMBTRACK
thumbtrack events (frequent events sent as the user drags the thumbtrack).
- EVT_COMMAND_SCROLL_THUMBRELEASE(func):
Process wxEVT_SCROLL_THUMBRELEASE
thumb release events.
- EVT_COMMAND_SCROLL_CHANGED(func):
Process wxEVT_SCROLL_CHANGED
end of scrolling events (MSW only).
- EVT_SLIDER(id, func):
Process
wxEVT_COMMAND_SLIDER_UPDATED
which is generated after any change of
wxSlider position in addition to one of the events above.
The difference between EVT_SCROLL_THUMBRELEASE and EVT_SCROLL_CHANGED
The EVT_SCROLL_THUMBRELEASE event is only emitted when actually dragging the thumb using the mouse and releasing it (This EVT_SCROLL_THUMBRELEASE event is also followed by an EVT_SCROLL_CHANGED event).
The EVT_SCROLL_CHANGED event also occurs when using the keyboard to change the thumb position, and when clicking next to the thumb (In all these cases the EVT_SCROLL_THUMBRELEASE event does not happen). In short, the EVT_SCROLL_CHANGED event is triggered when scrolling/ moving has finished independently of the way it had started. Please see the widgets sample ("Slider" page) to see the difference between EVT_SCROLL_THUMBRELEASE and EVT_SCROLL_CHANGED in action.
Appearance: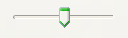 | 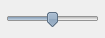 |  |
wxMSW appearance | wxGTK appearance | wxMac appearance |
- 参照:
- Events and Event Handling, wxScrollBar
Public Member Functions |
| wxSlider () |
| Default constructor.
|
| wxSlider (wxWindow *parent, wxWindowID id, int value, int minValue, int maxValue, const wxPoint &pos=wxDefaultPosition, const wxSize &size=wxDefaultSize, long style=wxSL_HORIZONTAL, const wxValidator &validator=wxDefaultValidator, const wxString &name=wxSliderNameStr) |
| Constructor, creating and showing a slider.
|
virtual | ~wxSlider () |
| Destructor, destroying the slider.
|
virtual void | ClearSel () |
| Clears the selection, for a slider with the wxSL_SELRANGE style.
|
virtual void | ClearTicks () |
| Clears the ticks.
|
bool | Create (wxWindow *parent, wxWindowID id, int value, int minValue, int maxValue, const wxPoint &point=wxDefaultPosition, const wxSize &size=wxDefaultSize, long style=wxSL_HORIZONTAL, const wxValidator &validator=wxDefaultValidator, const wxString &name=wxSliderNameStr) |
| Used for two-step slider construction.
|
virtual int | GetLineSize () const |
| Returns the line size.
|
virtual int | GetMax () const |
| Gets the maximum slider value.
|
virtual int | GetMin () const |
| Gets the minimum slider value.
|
virtual int | GetPageSize () const |
| Returns the page size.
|
virtual int | GetSelEnd () const |
| Returns the selection end point.
|
virtual int | GetSelStart () const |
| Returns the selection start point.
|
virtual int | GetThumbLength () const |
| Returns the thumb length.
|
virtual int | GetTickFreq () const |
| Returns the tick frequency.
|
virtual int | GetValue () const |
| Gets the current slider value.
|
virtual void | SetLineSize (int lineSize) |
| Sets the line size for the slider.
|
virtual void | SetPageSize (int pageSize) |
| Sets the page size for the slider.
|
virtual void | SetRange (int minValue, int maxValue) |
| Sets the minimum and maximum slider values.
|
virtual void | SetSelection (int startPos, int endPos) |
| Sets the selection.
|
virtual void | SetThumbLength (int len) |
| Sets the slider thumb length.
|
virtual void | SetTick (int tickPos) |
| Sets a tick position.
|
virtual void | SetTickFreq (int n) |
| Sets the tick mark frequency and position.
|
virtual void | SetValue (int value) |
| Sets the slider position.
|